Once you know about GitHub time tracking, what GitHub is, and how to use GitHub to host websites, you can move further and take full advantage of everything GitHub offers. One such thing is GitHub Actions.
In this GitHub Actions tutorial, weβll show you how to set up workflows to streamline software development tasks like CI/CD, testing, deployments, and automationβall natively inside your GitHub repository.
GitHub Actions offers several benefits, including automating various steps and phases in the software development cycle, such as creating pull requests, adding a new joiner to a repository, merging pull requests, and many more. Additionally, the feature allows users to set organization rules, for example, assigning developer permissions.
In this GitHub Actions tutorial, we will cover how to set up GitHub actions in your repository, how to set up your first GitHub Action, outline best practices to follow, and explore examples of how to use GitHub Actions.
What Are GitHub Actions and How Do They Work?
GitHub Actions allow you to automate software development workflows directly in your GitHub project. You can trigger automation based on events like pull requests, commits, or issue comments. Itβs a powerful way to build CI/CD pipelines without needing third-party tools.
Key Components of a GitHub Workflow
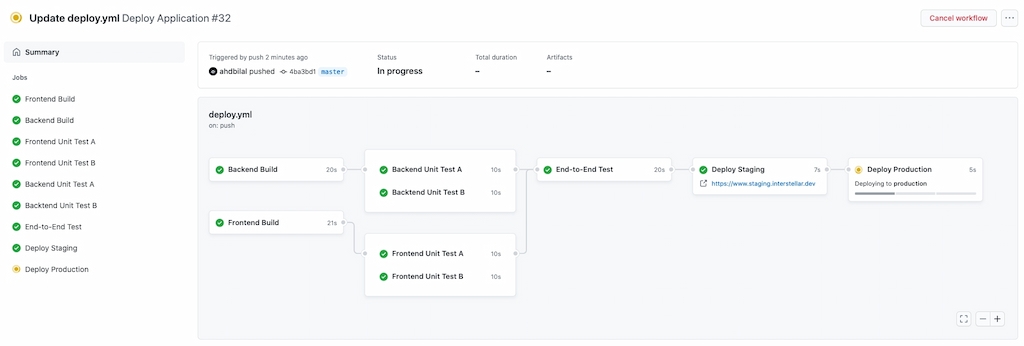
To understand how to use GitHub Actions, start by learning the core building blocks of a GitHub workflow:
- Workflows: YAML files that define automation logic
- Events: Triggers like push, pull_request, or custom
- Jobs: Groups of steps executed on a runner
- Actions: Reusable scripts to perform specific tasks
- Runners: Machines (hosted or self-hosted) that execute workflows
Events
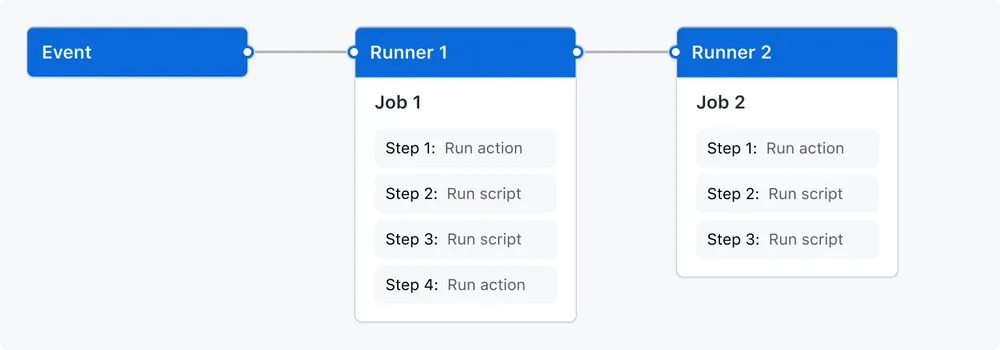
Events are defined triggers that start a workflow, such as creating a branch, opening a pull request, or commenting on an issue.
Jobs
Jobs are tasks that are executed in a workflow when an event is triggered. A workflow can have multiple jobs running in parallel.
Actions
Actions are used to perform complex tasks that you may import into workflows, such as sending a notification email. You can build your own actions or reuse open-source actions available on the GitHub marketplace.
Runner
A runner is a series of tasks that are executed in a workflow when triggered by an event. Each runner is responsible for executing a single job.
To summarize, GitHub Actions uses workflows, which are automated processes that are made up of jobs, events, actions and runners. Events are triggers that start a workflow, jobs are tasks executed in a workflow when an event is triggered, actions are used to perform complex tasks that are imported into workflows, and runners execute a single job. Now that weβve explored the components of a GitHub Action, letβs go through the set-up process.
How to Set Up GitHub Actions in a Repositor
To set up GitHub Actions, follow these steps:
- Create a folder: .github/workflows
- Add a YAML file like main.yml or github-actions-demo.yml
- Paste in your first basic GitHub Actions CI/CD pipeline script
name: GitHub Actions Demo on: [push] jobs: build: runs-on: ubuntu-latest steps: β run: echo βπ Triggered by a push event!β β name: Checkout code uses: actions/checkout@v3 |
Once committed, the workflow will be triggered by any push.
Popular GitHub Actions Examples You Can Try
Here are practical GitHub Actions examples to save time:
- β Read package.json file contents
- β Allow matrix job to fail without stopping build
- β Convert environment string to JSON
- β Welcome first-time contributors
- β Create releases with GitHub Release API
Youβll find all of these and more on the GitHub Actions Marketplace.
GitHub Actions Best Practices
Follow these GitHub Actions best practices for better performance and security:
- π΅οΈ Monitor every workflow for failures
- πββοΈ Keep steps and actions minimal
- π Ensure every repo includes a CI/CD pipeline
- β Set shorter workflow timeouts
- π Store sensitive info using secrets
- π Use descriptive names in jobs/steps
- π€ Explore and reuse actions from the GitHub Marketplace
π΅οΈββοΈ Monitor workflows
First and foremost, to ensure workflows are running correctly, make sure to monitor them as they run, and make changes if required.
πββοΈ Keep Actions minimal
Actionsβ have high bandwidth but are fast to complete. However, the longer an action takes to set up and then run, the more time you have to wait. For this reason, itβs best to keep Actions minimal.
GitHub Actions for CI/CD: Automate Build, Test & Deploy
Use GitHub Actions CI/CD to automate:
- Unit tests on each commit
- Linting and code formatting
- Deployment to Netlify, Vercel, or AWS
- Docker builds and pushes
- Automatic tagging and changelogs
CI/CD with GitHub is as simple as defining your pipeline in a workflow YAML.
β Set workflow timeouts
GitHub Actions kills workflows by default if they have not finished within six hours. While most workflows do not need that much time to complete, sometimes errors mean the job is killed after six hours have elapsed. Itβs best to set a shorter timeout, somewhere around 30 minutes should be enough time.
π Use Secrets to store sensitive information
Tokens or passwords should be stored using GitHub Secrets to ensure they stay secure. Secrets are encrypted variables created in an organization, repository, or repository environment to ensure sensitive information stays encrypted until it is used in a workflow.
π Use descriptive names
To ensure you understand your workflows fully, use descriptive names for jobs and steps to make the process easier to understand and modify if needed, as this will help you reduce the number of errors.
π€ Use the GitHub Actions marketplace
Use the GitHub Actions marketplace to discover hundreds of Actions created and published by other developers. Youβll find a wide selection of pre-built Actions that will save you time and effort when it comes to building efficient workflows.
π©βπ» Why Developers Use GitHub Actions for Project Automation
Whether youβre managing open source or enterprise-level codebases, developers use GitHub Actions to:
- Boost release frequency with fewer bugs
- Get feedback quickly with automated testing
- Collaborate more efficiently with built-in automation
- Eliminate manual processes from pull requests to deployment
π§ͺ Testing GitHub Actions: How to Monitor and Debug
- Use the βActionsβ tab in your GitHub repo to monitor real-time logs
- Rerun failed workflows manually
- Use echo steps to debug environment variables
- Check matrix logs for multiple environments
- Combine with GitHub Checks API for deep integration
β±οΈ Track Time in GitHub with Everhour Integration
Need to track how long your GitHub workflows take? Connect Everhour and GitHub for:
- Real-time GitHub time tracking
- Project budgeting & cost estimation
- Team workload visibility
- Reporting on tasks from GitHub issues or PRs
π Try Everhour for GitHub β itβs free to start and perfect for growing teams.
β GitHub Actions: Summary and Final Thoughts
- GitHub Actions brings powerful automation to your GitHub projects
- You can trigger workflows from commits, PRs, or issues
- Easily build CI/CD pipelines with reusable scripts
- Securely manage secrets and run tasks on GitHub-hosted runners
- Use GitHub Actions examples to simplify repetitive tasks
π Useful Links & Resources
- GitHub Actions Docs
- GitHub Actions Examples
- GitHub Marketplace
- Everhour GitHub Integration
- Jenkins vs GitHub Actions
- GitHub Copilot vs Microsoft Copilot
- Azure DevOps vs GitHub